Undetected ChromeDriver
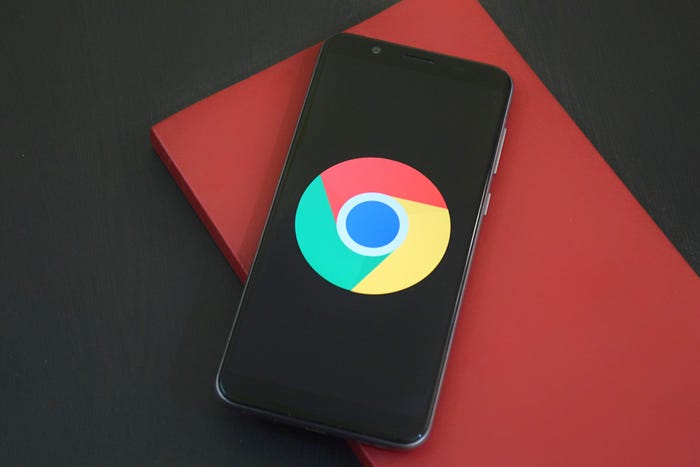
ChromeDriver
If you have used selenium in your web scraping or any other kind of automation projects, then you are definitely familiar with the WebDriver (or ChromeDriver most probably). A WebDriver is a protocol for controlling a browser while ChromeDriver and GeckoDriver are its implementations.
ChromeDriver is a small executable file that is required by your selenium script in order to control the chromium based browser (google chrome and brave are chromium based browsers).

The WebDriver protocol provides a very low-level specification, so it would be inefficient and hard to directly use the WebDriver. Selenium provides a high-level interface to use the WebDriver. Selenium has an API for multiple languages like Python, Java, Javascript etc.
The problem with using a normal ChromeDriver in your web scraping projects is that it is easily detectable. If the website is using an anti-bot measure like Cloudfare, Datadome etc, then you won’t be able to access the website and your IP can also get banned.
Undetected ChromeDriver
Using undetected ChromeDriver can give your bot some advantage and it can make your bot appear more like a human but keep in mind that it doesn’t guarantee success and it is not an alternative for proxies. It just makes your bot more authentic relative to a normal ChromeDriver because the normal ChromeDriver leaks a lot of information to the website which gets you caught.
How to use Undetected ChromeDriver
You need to install undetected-chromedriver
Python package before you can use it in your python. I encourage you to checkout its official documentation on how to do that.
Once you have installed the package, you can import and use it in your python code like this
import undetected_chromedriver as uc
import time
URL = 'https://nowsecure.nl'
driver = uc.Chrome()
driver.get(URL)
time.sleep(10)
The above code snippet shows the simplest use of the undetected ChromeDriver. But here is what happens under the hood.
uc.Chrome()
downloads the latest binary of the ChromeDriver and patches it in a way that fixes many ways an anti-bot system can detect our bot. You don’t need to manually download any ChromDriver binary but you have to make sure that the Chrome browser on your computer is up to date (for compatibility of ChromeDriver and Chrome browser). In case if your use case requires using a specific version of chrome browser , then you can specify that using an option called TARGET_VERSION
import undetected_chromedriver as uc
import time
uc.TARGET_VERSION = 85
URL = 'https://nowsecure.nl'
driver = uc.Chrome()
driver.get(URL)
time.sleep(10)
Now the undetected ChromeDriver will download a ChromeDriver binary for Chrome version 85 browser.
You can add options to the undetected ChromeDriver just like would to a normal ChromeDriver.
import undetected_chromedriver as uc
# Set Chrome Options
options = uc.ChromeOptions()
options.add_argument('--blink-settings=imagesEnabled=false')
# Create Undetected Chromedriver with Options
driver = uc.Chrome(options=options)
driver.get('https://nowsecure.nl')
But be careful while modifying the options because you might modify an option that the undetected ChromeDriver has already modified to make you less detectable and might end up doing its opposite.
Summary
Undetected ChromeDriver is a modified form of selenium ChromeDriver that automatically downloads the latest ChromeDriver binary and adds some necessary patches to it in order to avoid bot-detection.